useTrail
Overview
Creates multiple springs with a single config, each spring will follow the previous one. Use it for staggered animations.
Either: declaratively overwrite values to change the animation
If you re-render the component with changed props, the animation will update.
local springProps = {}
local length = #items
for index, item in ipairs(items) do
table.insert(springProps, {
transparency = if toggles[i] then 1 else 0,
})
end
local springs = RoactSpring.useTrail(length, springProps)
If you want the animation to run on mount, you can use from
to set the initial value.
local springProps = {}
local length = #items
for index, item in ipairs(items) do
table.insert(springProps, {
from = { transparency = item.transparency },
to = { transparency = if toggles[i] then 1 else 0 },
})
end
local springs = RoactSpring.useTrail(length, springProps)
Or: pass a function that returns values, and imperatively update using the api
You will get an API table back. It will not automatically animate on mount and re-render, but you can call api.start
to start the animation. Handling updates like this is generally preferred as it's more powerful. Further documentation can be found in Imperatives.
local length = #items
local springs, api = RoactSpring.useTrail(length, function(index)
return { transparency = items[index].transparency }
end)
-- Start animations
api.start(function(index)
return { position = UDim2.fromScale(0.5 * index, 0.16) }
end)
-- Stop all springs
api.stop()
Finally: apply styles to components
local contents = {}
for i = 1, 4 do
contents[i] = React.createElement("Frame", {
Position = springs[i].position,
Size = UDim2.fromScale(0.3, 0.3),
})
end
return contents
Properties
All properties documented in the common props apply.
By default, each spring will start 0.1 seconds after the previous one. You can override this by passing a delay
property.
-- Now each spring will start 0.2 seconds after the previous one
local springs, api = RoactSpring.useTrail(length, function(index)
return {
transparency = items[index].transparency,
delay = 0.2,
}
end)
You can also pass a delay
property to each spring individually.
-- The first spring will start 0.1 seconds after the previous one, the second 0.2 seconds, and so on
local springs, api = RoactSpring.useTrail(length, function(index)
return {
transparency = items[index].transparency,
delay = index * 0.1,
}
end)
Demos
Staggered list
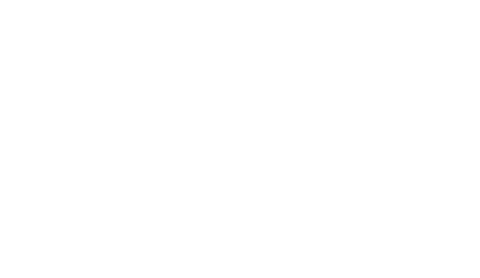
Staggered text
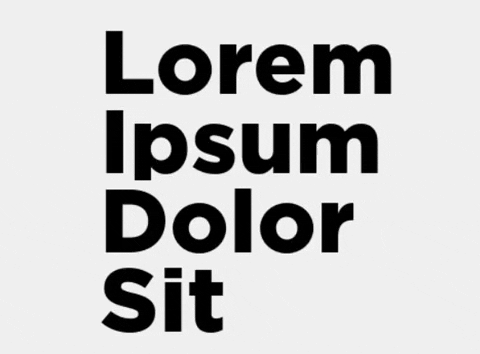
Trailing elements
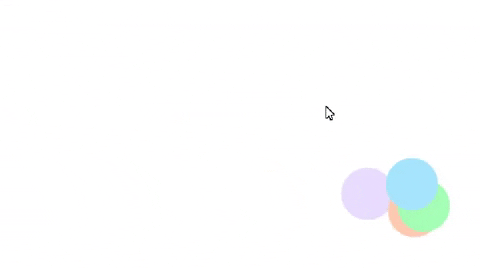